For this project, you will manage and submit your code on GitHub using GitHub Classroom. We have tutorials for them on our tutorials page.
Template Code
See the course website for the course at your home institution for links to the template and submission instructions.
Project Description
This project will introduce programming concepts in C++. The goal of the following activities is to prepare you to implement algorithms in C++. All the code will be compiled and executed in Replit.
Programming a Pocket Calculator
During lecture, we will go through how to program a pocket calculator in C++. You should implement the calculator in the provided template file, which is called main.cpp
. When your calculator is done, it should look something like this:
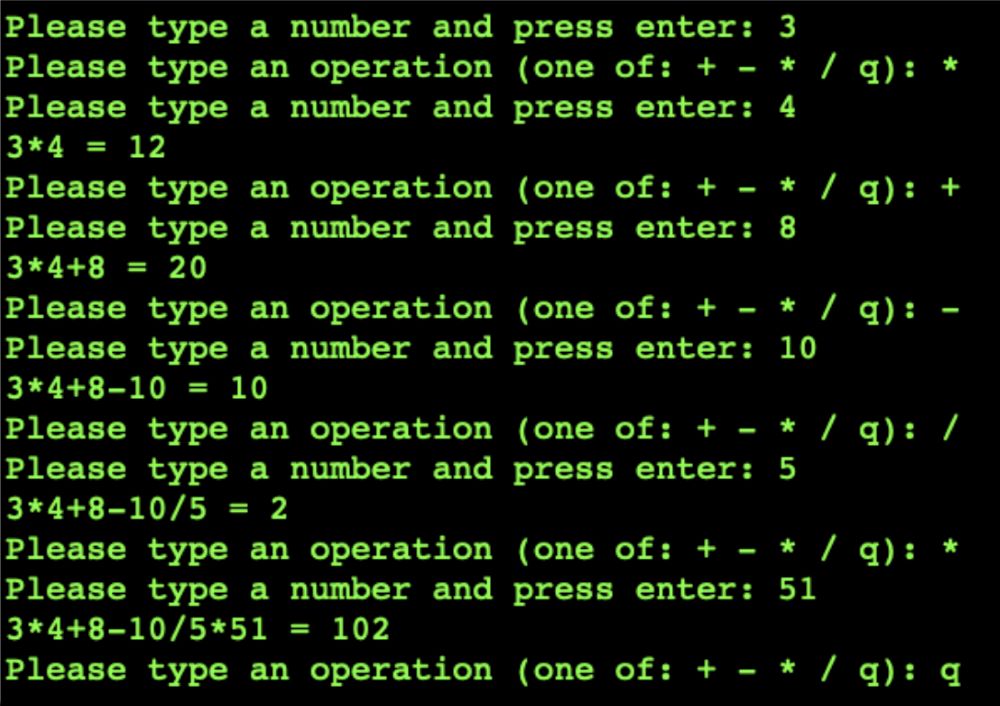
We will go through implementing these features to build up our pocket calculator together in the lectures (see Module 0 for links to lecture content). The list below summarizes features which your pocket calculator should implement. Use them as a checklist before submitting your assignment.
The project on Replit provides some automated tests, accessible in the left panel of the Replit project. These tests should all pass. However, these tests are not sufficient to ensure your code is working. You should test your code thoroughly with your own test cases.
-
P0.0:
In the file
LICENSE.txt
included in the project template, replace<COPYRIGHT HOLDER>
with your name. Replace<YEAR>
with the current year. - P0.1: Perform arithmetic operations for addition, subtraction, multiplication, and division for two numeric values and print the values, the operation, and their result to the screen.
- P0.2: Prompt a user to enter values and operators to the calculator. The program should take as input real valued numbers for the operands, and a character for the operator, and store these values in variables.
- P0.3: Create a function that computes a single arithmetic operation. The function should be given as input two floating point operands and a character that specifies the operation to be performed.
- P0.4: Iterate over an execution loop that performs an infinite number of subsequent arithmetic operations given from user input, until the user specifies the operator 'q' for quit. Output the result accumulated over subsequently performed operations.
- P0.5: Check whether the user has provided valid input for the operator. If the input is invalid, display an error message and request the input again.
Advanced Extensions
Advanced extensions are optional features which you may complete to explore this assignment further.
- Advanced Extension P0.i: Enable the user to undo their last operation by specifying 'u' as the operator.
- Advanced Extension P0.ii: Enable the user to clear the calculator by specifying 'c' as the operator.
- Advanced Extension P0.iii: Compute the final result of the user specified equation using the Order of Operations.
- Advanced Extension P0.iv: Enable the user to store and load their current set of operations through a file named "resultcalc.txt" such that this equation can be used across multiple program executions.